Create And Use Unit Tests with JavaScript
Unit Tests in JavaScript
In this blog post we are going to create a brand-new NodeJS project with Jasmine to run some unit tests with JavaScript.
Set up a new NodeJS Project
First make sure you have NodeJS installed. You can find the installer and resources here. You can check if the installation was successful by typing node in your terminal. When you get no error after pressing enter you are ready to move on, otherwise double-check the installation.
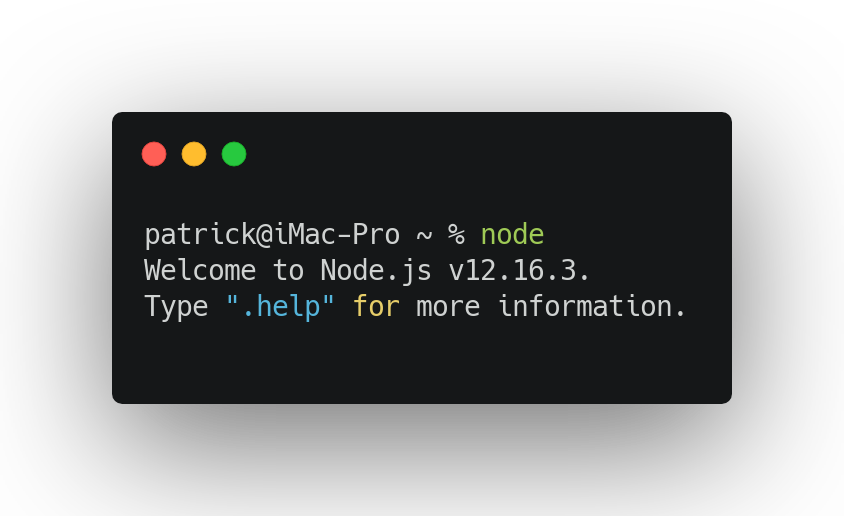
Move to your folder of choice and type node init. This command initializes a new NodeJS project. Basically, it guides you through the process of creation the package.json file. This file holds all your project details like title, author, git etc. and your Node Packages, which you will eventually show up in your node_modules folder. No worries, if you are not familiar with all of this, you will find plenty of resources online about it.
Adding Jasmine for Unit Tests
Alright, now it’s time to set up our project. See my package.json below for comparison. After this we have to add one more dependency. It’s the Jasmine testing Library. With npm install -save--dev jasmine the dependency is only available in our development environment. This makes no real difference now, but keep in mind when you set up a production build all dev dependencies are ignored. You simply don‘t need a testing framework on your production website for example.
Perfect - the last setup step is to set up Jasmine properly. With jasmine init you can initialize jasmine. You should now see a spec folder. In JavaScript usually all test files have a ...spec.js ending. Some parts of the community put all their tests into a dedicated spec folder, and some not. Just keep that in mind, so that you know what’s going on when you see a spec folder with many spec.js files. Finally, hop back into the package.json file to change the test script to { „test“ : „jasmine“}. That ensures when we call npm test that jasmine is fired up.
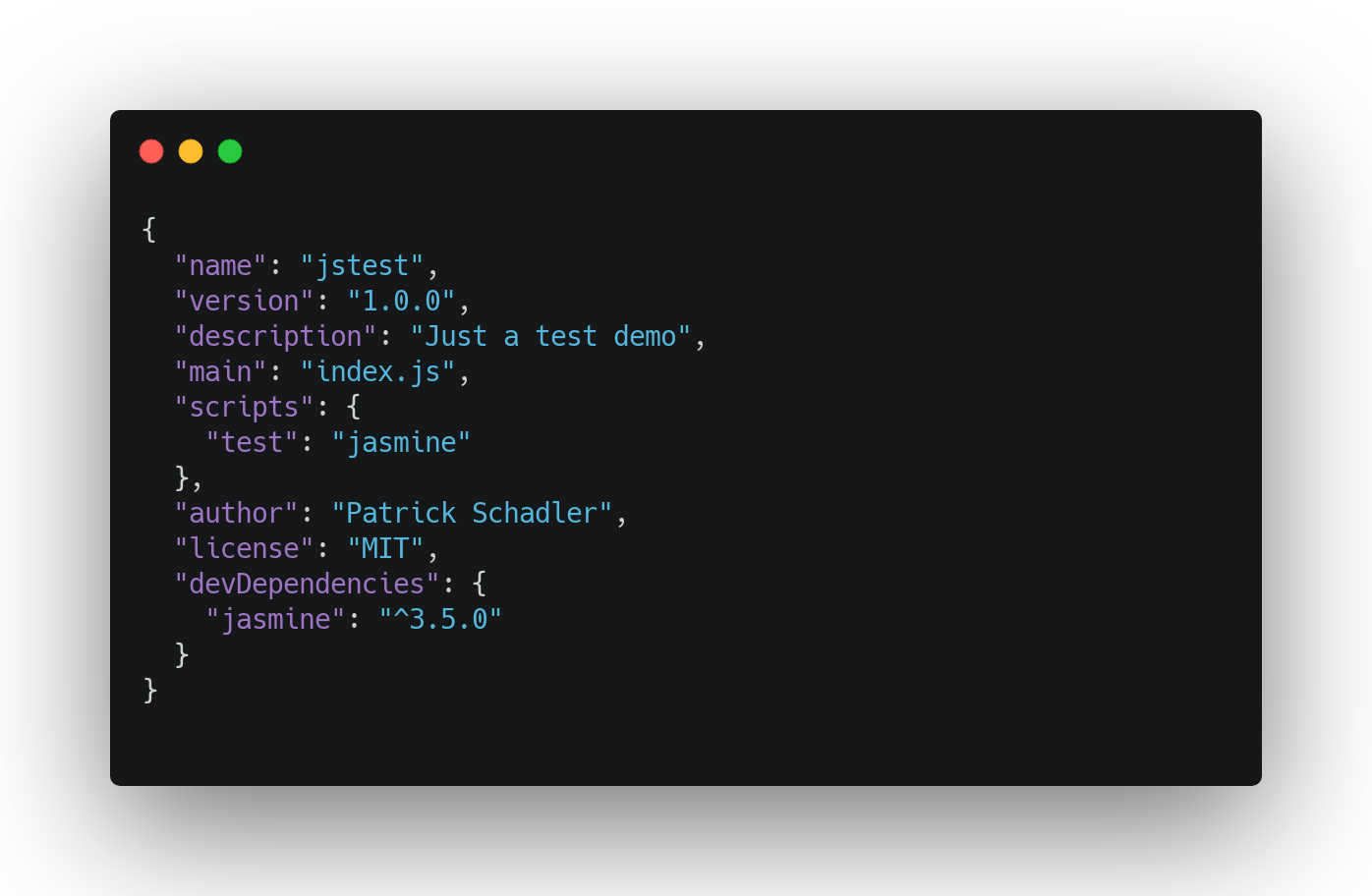
Writing Tests
Let us create an index.spec.js file within the spec folder. This file will hold all our tests for this tutorial. Now, to see if our setup is working as expected copy the skeleton test from below.
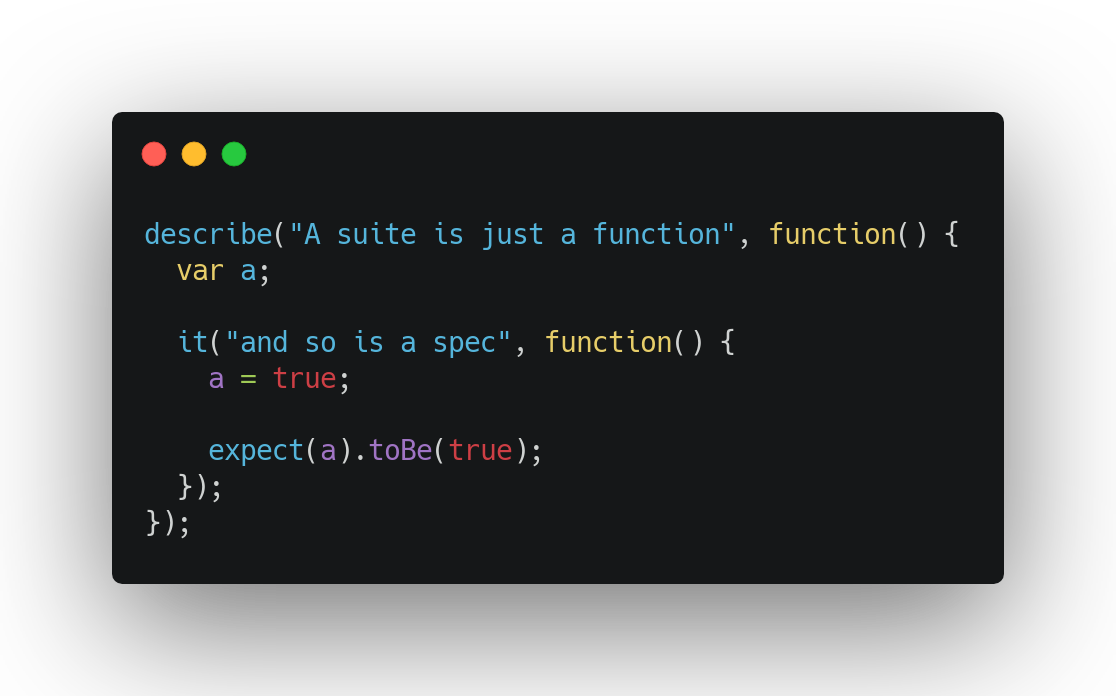
This test just compares if the variable a, which is set to true, is actually true. Not the greatest test of all time, but if you run the test and everything succeeds it shows that our setup is working.
To test a simple function that adds two numbers create an index.js file. I know, not the best naming convention, but we want to KISS (Keep it simple & stupid) here.
This file holds an arrow function which adds two arguments and returns the sum.
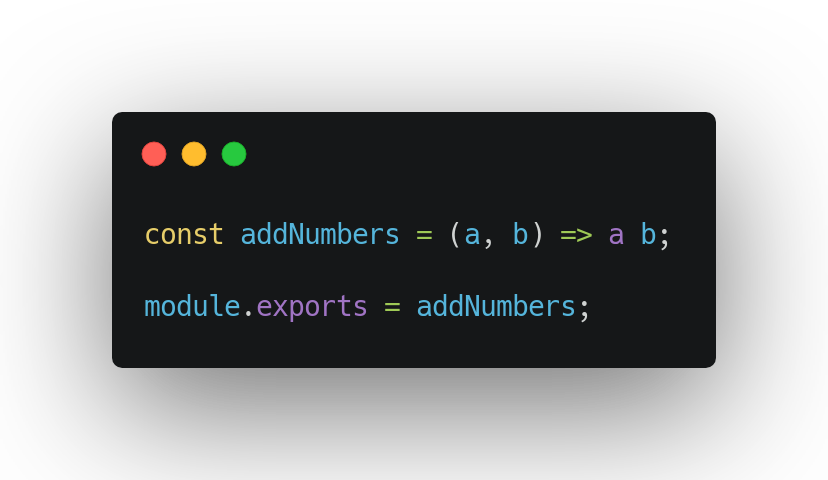
By writing this you can already think about some test cases. Surely the obvious one is when you add two numbers, but what happens when you add two strings, Boolean, objects, arrays etc? You already see, you will end up writing many tests for just a simple function like this.
Now, back to the spec file. At first, we add another it function for our test. Let’s call it „2 2 should be 4“. In this it function we are calling the addNumbers function from our index.js file. To get this running we need to import the index.js_ properly. Add a const addNumber = require("../../index.js) at the top of the spec file. Make sure you change your import accordingly to your file location.
Assert the expected and the actual values
Finally, we can call the addNumbers function with two arguments, 2 and 2, in our case. Let's store the result in a const and assert it. For assertion, we are going to use the expect function. It lets you define the expected and actual result. When both matches everything is fine, when they are different the test is failing. You can play around with it to see how it works. For now, we write expect(result).toBe(4) and hit npm test in the terminal. When everything is working as expected the test run should succeed and the terminal should display no errors.
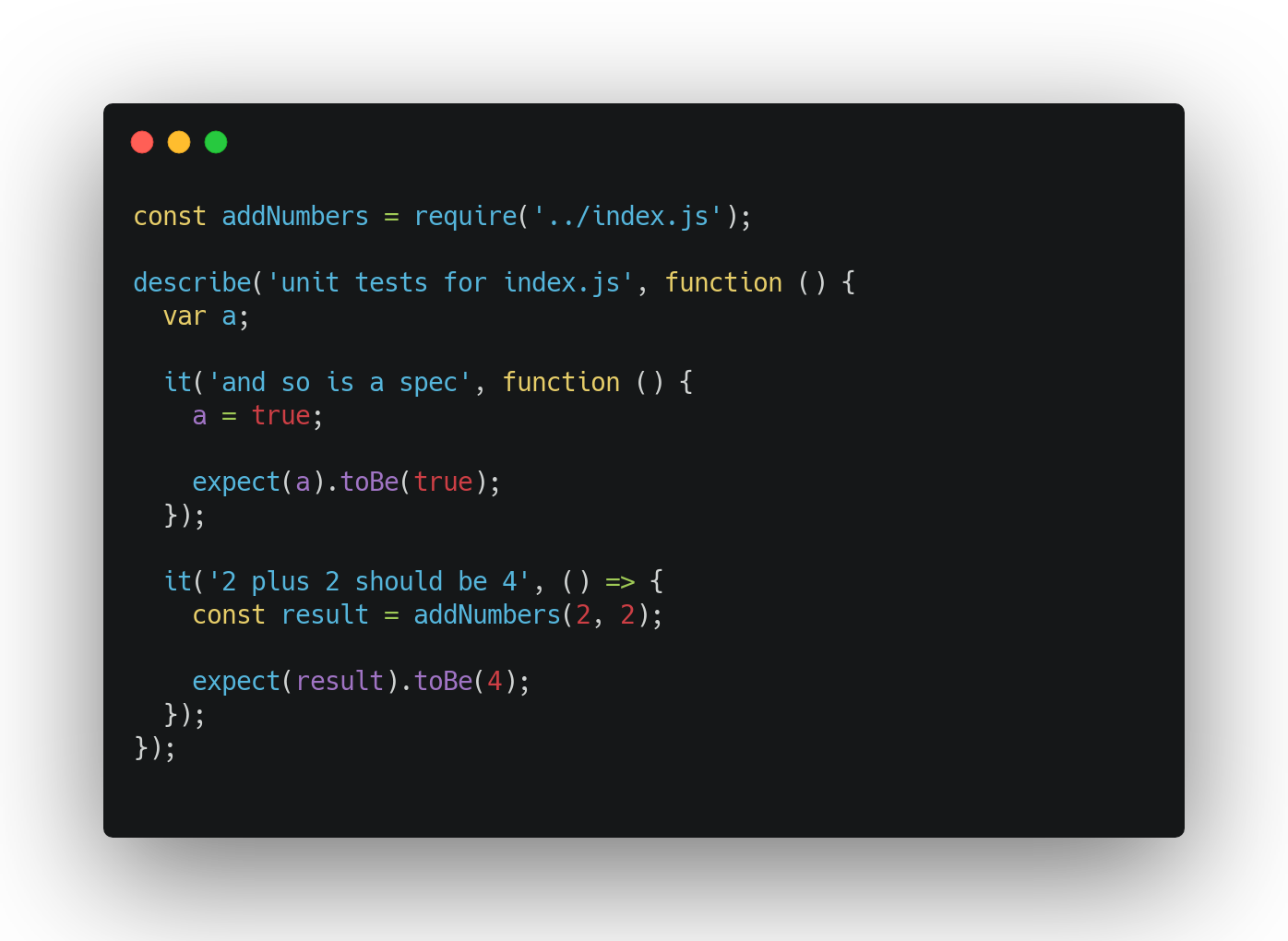
Conclusion
That's it. Writing Unit Tests in JavaScript is easy when you understand the fundamentals. JavaScript being a dynamic language makes writing unit tests even easier, but I would highly recommend that you check our TypeScript as well. Surely this tutorial only covered a new NodeJS project and Jasmine, but the principles of writing unit tests are always the same, no matter if you are using Jest with ReactJS or any backend language.
Do you have something to add? Or did you miss anything? Whatever it is, drop a comment below and check out my YouTube video about this very topic HERE. Want to create a Web Service with C# then read this post.