Creating A Web Service With C# and Visual Studio
Creating A Web Service With C# and Visual Studio is probably simpler than many people actually think. In this example I will cover the creation of a Windows Communication Foundation (WCF) Service and how I test those services with tools like SOAP UI - which by the way I highly recommend for any web developer.
1. Create a C# Project
In my example I create a C# Web Project with the predefined Model View Controller (MVC) project type. I won't use the full potential of the MVC type, but however I do like the design pattern itself and it's very easy to add an administration panel or something like that later on. So for now, don't get too much confused by that :-).
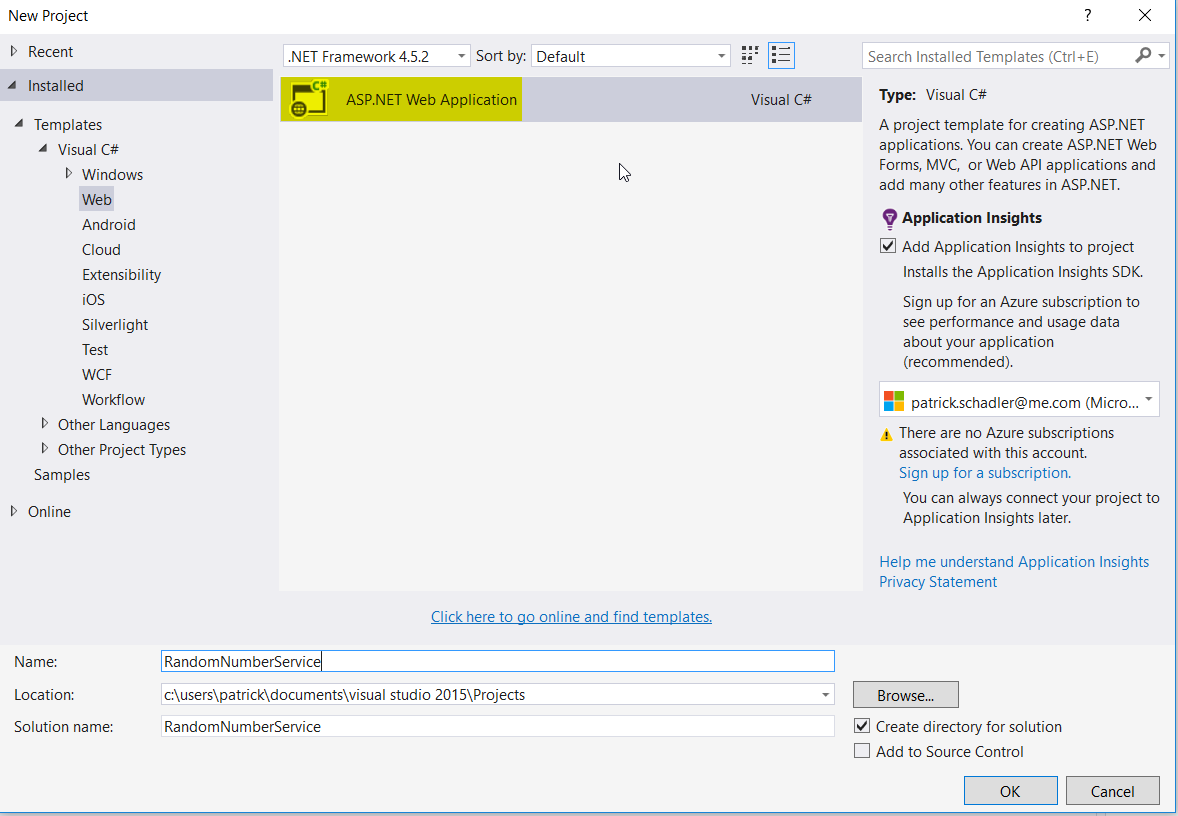
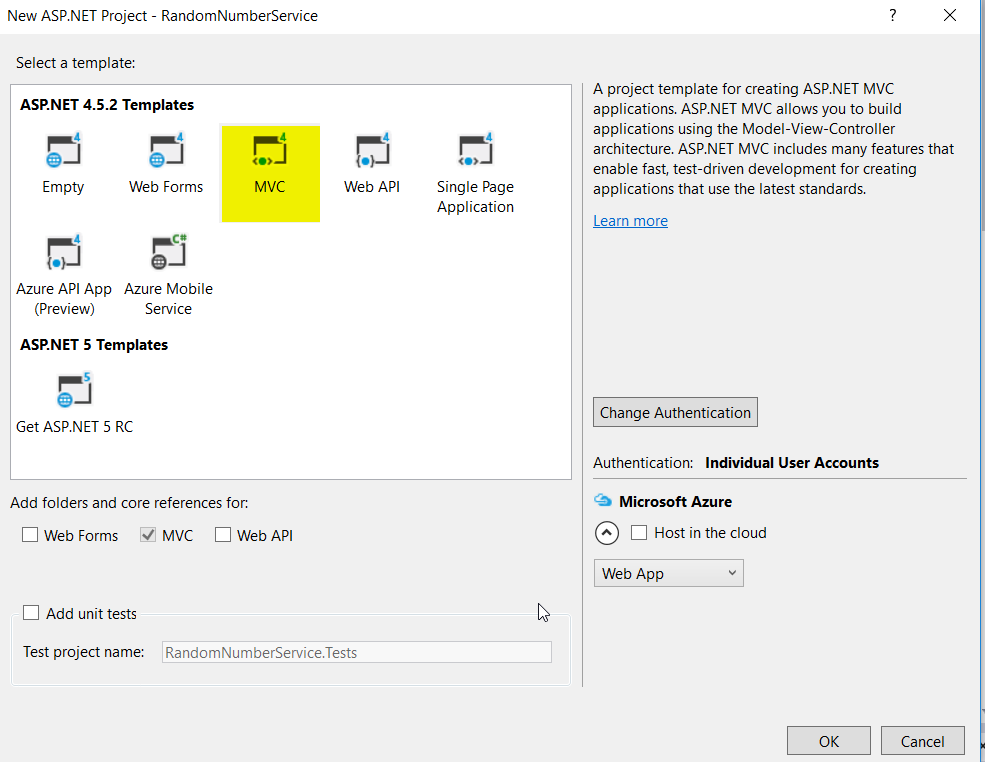
2. Add a WCF Service
By Adding a WCF Service Visual Studio automatically generates an interface and an implementation of that interface. For our example we remove the default doWork() method and add an new GetRandomNumbers() method - which will have a return value - the NumberModel.
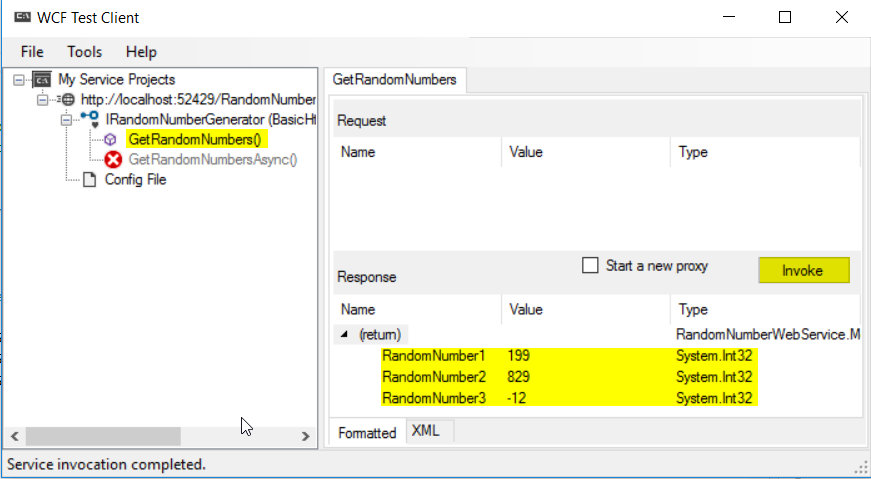
[ServiceContract]
public interface IRandomNumberGenerator
{
[OperationContract]
NumberModel GetRandomNumbers();
}
The NumberModel - as the name suggest - will be used as a DataModel to store three different random numbers. So we put a new .cs file in the Models folder and name it NumberModel.cs.
After me made sure that the NumberModel is public we add three properties RandomNumber1, RandomNumber2 and RandomNumber3 with the public access modifier and they are of type integer.
public class NumberModel
{
public int RandomNumber1 { get; set; }
public int RandomNumber2 { get; set; }
public int RandomNumber3 { get; set; }
}
In our implementation of GetRandomNumbers() we now add the code for random number generation, fill a NumberNodel object and return it.
The Service caller now gets our newly created RandomNumber model as a result when calling the GetRandomNumbers() method.
public class RandomNumberGenerator : IRandomNumberGenerator
{
NumberModel IRandomNumberGenerator.GetRandomNumbers()
{
var random = new Random();
var numbermodel = new NumberModel
{
RandomNumber1 = random.Next(-500,1000),
RandomNumber2 = random.Next(-500, 1000),
RandomNumber3 = random.Next(-500, 1000),
};
return numbermodel;
}
}
3. Test the Service with the integrated WCF Test Client
After you run the application Visual Studio automatically detects a WCF Service and starts the WCF Test Client. Within this Client you can invoke a service call - and you'll see the results as shown in the image below.
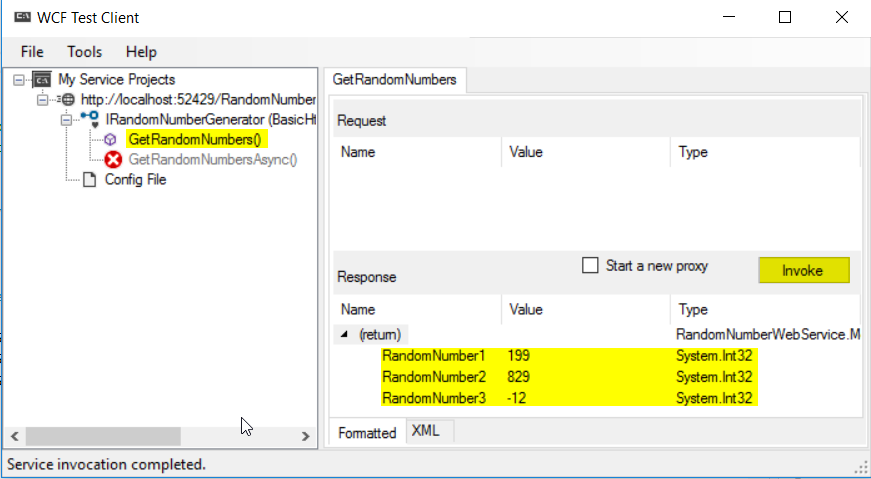
As I said in the beginning I prefer SOAP UI as my go to web service testing tool. It can handle various types of services, has a lot of configuration settings and never let me down so far.
If you want to add a new service, in our case we click new SOAP project, enter our local service url and add ?wsdl to the url and SOAP UI generates all available requests for us. If he hit now the execute button a request is send to the service and on the right side we'll se the result of the service call.
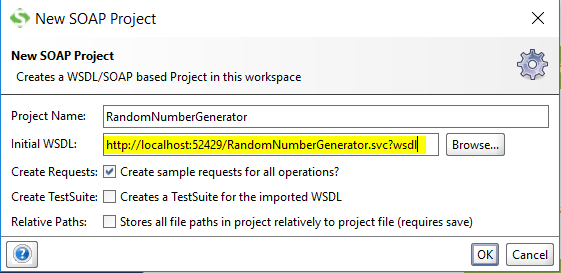
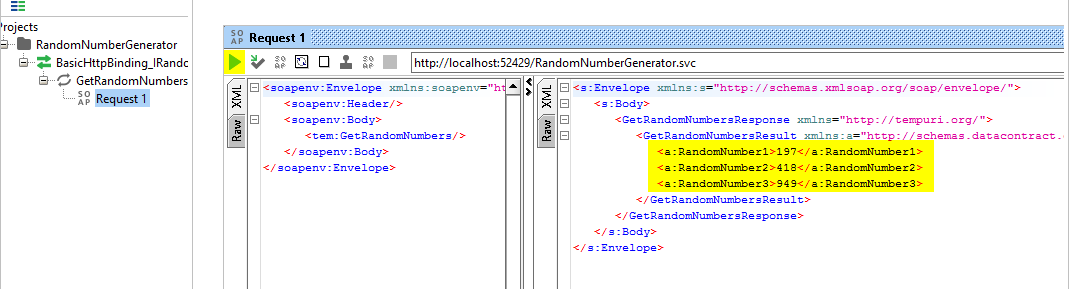
You also find a highly recommended video tutorial here: